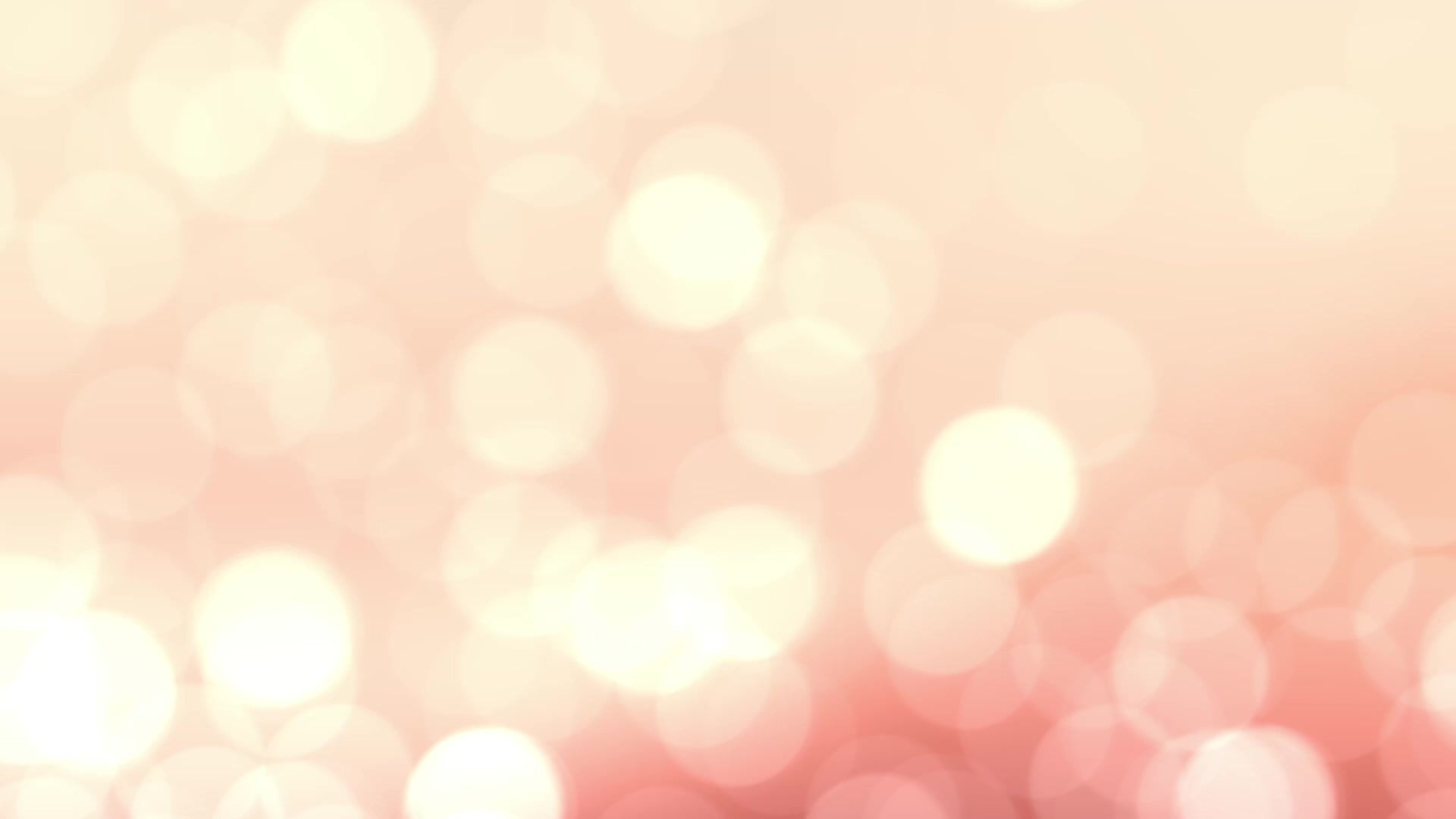
Board #3: LED
These are the codes for Arduino board that is responsible for LED strip flashing:
[Take note that FastLED.h library must be downloaded to use these code for LED strip flashing]
#include <FastLED.h>
#define NUM_LEDS 60
#define DATA_PIN 7
#define CLOCK_PIN 13
CRGB leds[NUM_LEDS];
void setup() {
LEDS.addLeds<WS2811,DATA_PIN,GRB>(leds,NUM_LEDS);
LEDS.setBrightness(200);
pinMode(A3, INPUT); //MSB
pinMode(A4, INPUT); //LSB
//01: Blink every 1 sec
//10: Cylon
}
void fadeall() { for(int i = 0; i < NUM_LEDS; i++) { leds[i].nscale8(250); } }
void LED_Blink(){
for(int i=0; i<NUM_LEDS;i++)
{
leds[i] = CRGB::Red;
}
FastLED.show();
delay(500);
for(int i=0; i<NUM_LEDS;i++)
{
leds[i] = CRGB::Black;
}
FastLED.show();
delay(500);
}
void LED_Cylon(){
static uint8_t hue = 0;
Serial.print("x");
// First slide the led in one direction
for(int i = 0; i < NUM_LEDS; i++) {
// Set the i'th led to red
leds[i] = CHSV(hue++, 255, 255);
// Show the leds
FastLED.show();
// now that we've shown the leds, reset the i'th led to black
// leds[i] = CRGB::Black;
fadeall();
// Wait a little bit before we loop around and do it again
delay(10);
}
}
void loop() {
if(digitalRead(A3)==LOW && digitalRead(A4)==HIGH)
{
LED_Blink();
}
if(digitalRead(A3)==HIGH && digitalRead(A4)==LOW)
{
LED_Cylon();
}
if(digitalRead(A3)==LOW && digitalRead(A4)==LOW)
{
for(int i=0; i<NUM_LEDS;i++)
{
leds[i] = CRGB(0,0,0);
}
}
}